原创首发
阳光沙滩博客系统-SpringJap的增删改查练习
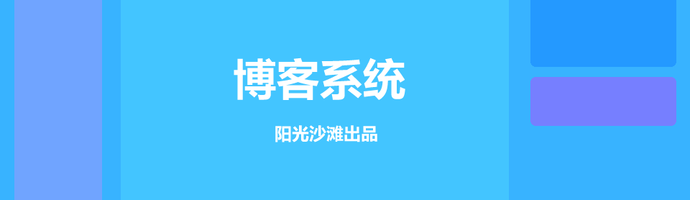
以Label为例子
Label的Dao
public interface LabelDao extends JpaRepository<Label, String>, JpaSpecificationExecutor<Label> {
@Modifying
int deleteOneById(String id);
@Modifying
@Query(value = "DELETE FROM `tb_labels` WHERE id = ?", nativeQuery = true)
int customDeleteLabelById(String id);
/**
* 根据ID查找一个标签
*
* @param id
* @return
*/
Label findOneById(String id);
Label findOneByName(String name);
}
添加
@PostMapping("/label")
public ResponseResult addLabel(@RequestBody Label label) {
//判断数据是否有效
//补全数据
label.setId(idWorker.nextId() + "");
label.setCreateTime(new Date());
label.setUpdateTime(new Date());
//保存数据
labelDao.save(label);
return ResponseResult.SUCCESS("测试标签添加成功");
}
删除
@DeleteMapping("/label/{labelId}")
public ResponseResult deleteLabel(@PathVariable("labelId") String labelId) {
int deleteResult = labelDao.customDeleteLabelById(labelId);
log.info("deleteResult == > " + deleteResult);
if (deleteResult > 0) {
return ResponseResult.SUCCESS("删除标签成功");
} else {
return ResponseResult.FAILED("标签不存在");
}
}
修改/更新
@PutMapping("/label/{labelId}")
public ResponseResult updateLabel(@PathVariable("labelId") String labelId, @RequestBody Label label) {
Label dbLabel = labelDao.findOneById(labelId);
if (dbLabel == null) {
return ResponseResult.FAILED("标签不存在");
}
dbLabel.setCount(label.getCount());
dbLabel.setName(label.getName());
dbLabel.setUpdateTime(new Date());
labelDao.save(dbLabel);
return ResponseResult.SUCCESS("修改成功");
}
查询
@GetMapping("/label/{labelId}")
public ResponseResult getLabelById(@PathVariable("labelId") String labelId) {
Label dbLabel = labelDao.findOneById(labelId);
if (dbLabel == null) {
return ResponseResult.FAILED("标签不存在");
}
return ResponseResult.SUCCESS("获取标签成功").setData(dbLabel);
}
分业查询
@GetMapping("/label/list/{page}/{size}")
public ResponseResult listLabels(@PathVariable("page") int page, @PathVariable("size") int size) {
if (page < 1) {
page = 1;
}
if (size <= 0) {
size = Constants.DEFAULT_SIZE;
}
Sort sort = new Sort(Sort.Direction.DESC, "createTime");
Pageable pageable = PageRequest.of(page - 1, size, sort);
Page<Label> result = labelDao.findAll(pageable);
return ResponseResult.SUCCESS("获取成功").setData(result);
}
条件查询
@GetMapping("/label/search")
public ResponseResult doLabelSearch(@RequestParam("keyword") String keyword, @RequestParam("count") int count) {
List<Label> all = labelDao.findAll(new Specification<Label>() {
@Override
public Predicate toPredicate(Root<Label> root, CriteriaQuery<?> criteriaQuery, CriteriaBuilder cb) {
Predicate namePre = cb.like(root.get("name").as(String.class), "%" + keyword + "%");
Predicate countPre = cb.equal(root.get("count").as(Integer.class), count);
Predicate and = cb.and(namePre, countPre);
return and;
}
});
if (all.size() == 0) {
return ResponseResult.FAILED("结果为空");
}
return ResponseResult.SUCCESS("查找成功").setData(all);
}