根据framlayout注入fragment实现切换ui不显示的问题
我要在第一个图的framlayout里面注入xml为图2的fragment, ui不展示图2的内容 debug了 switchFragment方法是正常走完了的,但是ui确实不展示图2的内容。
图1
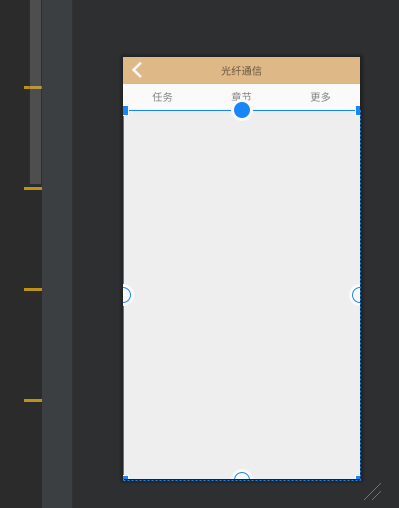
图2
public class CoursesLearnedActivity extends BaseActivity{
private CourseTaskFragment mCourseTaskFragment;
private CourseChapterFragment mCourseChapterFragment;
private CourseMoreFragment mCourseMoreFragment;
@BindView(R.id.course_name)
public TextView mCourseName;
@BindView(R.id.course_task)
public TextView mCourseTask;
@BindView(R.id.course_chapter)
public TextView mCourseChapter;
@BindView(R.id.course_more)
public TextView mCourseMore;
@Override
protected void initPresenter() {
mCourseTask.setOnClickListener(v -> {
switchFragment(mCourseTaskFragment);
LogUtils.d(this, "切换到任务");
});
mCourseChapter.setOnClickListener(v -> {
switchFragment(mCourseChapterFragment);
LogUtils.d(this, "切换到章节");
});
mCourseMore.setOnClickListener(v -> {
switchFragment(mCourseMoreFragment);
LogUtils.d(this, "切换到更多");
});
}
private FragmentManager mFm;
@Override
protected void initView() {
Intent intent = new Intent();
String courseName = intent.getStringExtra("course_name");
mCourseName.setText(courseName);
mCourseTaskFragment = new CourseTaskFragment();
mCourseChapterFragment = new CourseChapterFragment();
mCourseMoreFragment = new CourseMoreFragment();
mFm = getSupportFragmentManager();
switchFragment(mCourseTaskFragment);
}
@Override
protected int getLayoutResId() {
return R.layout.activity_courses_learned;
}
/**
* 上一次显示的fragment
*/
private BaseFragment lastOneFragment = null;
private void switchFragment(BaseFragment targetFragment) {
//如果上一个fragment跟当前切换的fragment是同一个,那么不需要切换
if (lastOneFragment == targetFragment) {
return;
}
//修改成add和hide的方式来控制Fragment的切换
FragmentTransaction fragmentTransaction = mFm.beginTransaction();
if (!targetFragment.isAdded()) {
fragmentTransaction.add(R.id.course_item_container, targetFragment);
} else {
fragmentTransaction.show(targetFragment);
}
if (lastOneFragment != null) {
fragmentTransaction.hide(lastOneFragment);
}
lastOneFragment = targetFragment;
fragmentTransaction.commit();
}
}
图1xml文件
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<FrameLayout
android:id="@+id/course_item_container"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_below="@+id/view_title_bar"
android:background="#EFEEEE" />
<LinearLayout
android:id="@+id/view_title_bar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@+id/constraintLayout"
android:layout_gravity="center_vertical"
android:orientation="horizontal">
<TextView
android:id="@+id/course_task"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:gravity="center"
android:padding="10dp"
android:text="任务"
android:textColor="@color/gray"
android:textSize="18sp" />
<TextView
android:id="@+id/course_chapter"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:gravity="center"
android:padding="10dp"
android:text="章节"
android:textColor="@color/gray"
android:textSize="18sp" />
<TextView
android:id="@+id/course_more"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:gravity="center"
android:padding="10dp"
android:text="更多"
android:textColor="@color/gray"
android:textSize="18sp" />
</LinearLayout>
<RelativeLayout
android:id="@+id/constraintLayout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@color/burlywood"
android:orientation="horizontal">
<ImageView
android:id="@+id/ic_back"
android:layout_width="30dp"
android:layout_height="30dp"
android:layout_centerVertical="true"
android:layout_marginStart="10dp"
android:src="@mipmap/back" />
<TextView
android:id="@+id/course_name"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:ellipsize="end"
android:gravity="center"
android:padding="@dimen/dp_10"
android:text="光纤通信"
android:textSize="18sp" />
</RelativeLayout>
</RelativeLayout>
图2 java文件
public class CourseTaskFragment extends BaseFragment {
@Override
protected int getRootViewResId() {
return R.layout.fragment_course_task;
}
@Override
protected void initListener() {
super.initListener();
}
}
图2 xml文件
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<androidx.constraintlayout.widget.ConstraintLayout
android:id="@+id/course_discuss"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@color/white"
android:gravity="center_vertical"
android:orientation="horizontal"
android:paddingTop="5dp"
android:paddingBottom="5dp">
<ImageView
android:id="@+id/task_cover"
android:layout_width="60dp"
android:layout_height="60dp"
android:layout_marginLeft="5dp"
android:scaleType="fitXY"
android:src="@mipmap/discuss"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<ImageView
android:layout_width="30dp"
android:layout_height="30dp"
android:layout_marginStart="10dp"
android:layout_marginEnd="10dp"
android:src="@mipmap/arrows"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/course_discuss_title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="10dp"
android:layout_marginEnd="19dp"
android:padding="15dp"
android:text="讨论"
android:textSize="18sp"
android:textStyle="bold"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintStart_toEndOf="@+id/task_cover"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
<View
android:id="@+id/view"
android:layout_width="match_parent"
android:layout_height="1dp"
android:background="@color/gray" />
<androidx.constraintlayout.widget.ConstraintLayout
android:layout_width="match_parent"
android:id="@+id/course_exam"
android:layout_height="70dp"
android:background="@color/white"
android:gravity="center_vertical"
android:orientation="horizontal"
android:paddingTop="5dp"
android:paddingBottom="5dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/view">
<ImageView
android:id="@+id/image2"
android:layout_width="60dp"
android:layout_height="60dp"
android:layout_marginLeft="5dp"
android:scaleType="fitXY"
android:src="@mipmap/exam"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<ImageView
android:layout_width="30dp"
android:layout_height="30dp"
android:layout_marginStart="10dp"
android:layout_marginEnd="10dp"
android:src="@mipmap/arrows"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/course_exam_title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="10dp"
android:layout_marginEnd="19dp"
android:padding="15dp"
android:text="作业/考试"
android:textSize="18sp"
android:textStyle="bold"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintStart_toEndOf="@+id/image2"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
</LinearLayout>
1.首先确定你的framelayout位置对不对 (建议你的相对布局还是从上往下写好 第一次看好乱[捂脸]) 用个view代替加个背景看看 排除xml的问题
2.baseFragment 没看出内容 如果它能正常使用的话 你可以先用
这些代码去静态加载你的fragment 排除是fragment问题