java 利用httpurlconnection和io流爬取音乐,循环爬取就会出现io流读不完,文件爬取不完全
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
import java.io.*;
import java.net.*;
import java.util.*;
public class NetRequest_Music {
HashMap<String, String> getMusicList(String serchkey) {
try {
serchkey = URLEncoder.encode(serchkey, "UTF-8");
String stringUrl = "http://www.kuwo.cn/api/www/search/searchMusicBykeyWord?key=" + serchkey + "&pn=1&rn=30&httpsStatus=1&reqId=7351da11-f940-11ec-97d0-5b5ac2228900";
URL url = new URL(stringUrl);
System.out.println(url);
HttpURLConnection httpURLConnection = (HttpURLConnection) url.openConnection();
httpURLConnection.setRequestMethod("GET");
httpURLConnection.setRequestProperty("Cookie", "_ga=GA1.2.1199988116.1656681561; _gid=GA1.2.1623023781.1656681561; _gat=1; Hm_lvt_cdb524f42f0ce19b169a8071123a4797=1656681561; Hm_lpvt_cdb524f42f0ce19b169a8071123a4797=1656681561; kw_token=6H8JN2KUMWF");
httpURLConnection.setRequestProperty("Referer", "http://www.kuwo.cn/search/list?key=徐良");
httpURLConnection.setRequestProperty("csrf", "6H8JN2KUMWF");
httpURLConnection.setRequestProperty("User-Agent", "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/103.0.0.0 Safari/537.36");
httpURLConnection.setConnectTimeout(100000);
httpURLConnection.setReadTimeout(100000);
httpURLConnection.setDoInput(true);
httpURLConnection.connect();
if (httpURLConnection.getResponseCode() == HttpURLConnection.HTTP_OK) {
System.out.println("------>responsecode" + 200);
InputStream inputStream = httpURLConnection.getInputStream();
byte[] blist = new byte[1024];
StringBuffer stringBuffer = new StringBuffer();
int s;
while ((s = inputStream.read(blist)) != -1) {
stringBuffer.append(new String(blist, 0, s));
}
String musicurl = stringBuffer.toString();
JSONObject jsonObject = JSON.parseObject(musicurl);
JSONArray infolist = jsonObject.getJSONObject("data").getJSONArray("list");
HashMap<String, String> ridWithSongHashMap = new HashMap<>();
int i = 0;
for (Object j : infolist
) {
JSONObject jj = (JSONObject) j;
ridWithSongHashMap.put(jj.getString("rid"), jj.getString("name"));
}
inputStream.close();
return ridWithSongHashMap;
} else {
System.out.println(httpURLConnection.getResponseCode() + "------->连接失败");
}
} catch (IOException unsupportedEncodingException) {
unsupportedEncodingException.printStackTrace();
}
return null;
}
String searchSongUrl(String mid) {
try {
URL url = new URL("https://www.kuwo.cn/api/v1/www/music/playUrl?format=mp3&mid=" + mid + "&response=url&type=convert_url3&br=128kmp3&from=web");
HttpURLConnection httpURLConnection = (HttpURLConnection) url.openConnection();
httpURLConnection.setRequestMethod("GET");
httpURLConnection.setRequestProperty("User-Agent", "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/75.0.3770.100 Safari/537.36");
httpURLConnection.setRequestProperty("Cookie", "Hm_lvt_cdb524f42f0ce19b169a8071123a4797=1577029678,1577034191,1577034210,1577076651; Hm_lpvt_cdb524f42f0ce19b169a8071123a4797=1577080777; kw_token=RUJ53PGJ4ZD");
httpURLConnection.setRequestProperty("Referer", "http://www.kuwo.cn/search/list?key=%E5%91%A8%E6%9D%B0%E4%BC%A6");
httpURLConnection.setConnectTimeout(100000);
httpURLConnection.setReadTimeout(100000);
httpURLConnection.setDoInput(true);
httpURLConnection.connect();
if (httpURLConnection.getResponseCode() == HttpURLConnection.HTTP_OK) {
System.out.println("------>responsecode" + 200);
InputStream inputStream = httpURLConnection.getInputStream();
byte[] blist = new byte[1024];
StringBuffer stringBuffer = new StringBuffer();
int s;
while ((s = inputStream.read(blist)) != -1) {
stringBuffer.append(new String(blist, 0, s));
}
String musicurl = stringBuffer.toString();
JSONObject jsonObject = JSON.parseObject(musicurl);
System.out.println(jsonObject.getJSONObject("data").getString("url"));
inputStream.close();
return url.toString();
} else {
System.out.println(httpURLConnection.getResponseCode() + "------->连接失败");
}
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
static void downloadMusic(String musicurl, String musicname) {
try {
URL url = new URL(musicurl);
HttpURLConnection httpURLConnection = (HttpURLConnection) url.openConnection();
httpURLConnection.setRequestMethod("GET");
httpURLConnection.setConnectTimeout(100000);
httpURLConnection.setReadTimeout(100000);
httpURLConnection.setDoInput(true);
httpURLConnection.connect();
if (httpURLConnection.getResponseCode() == HttpURLConnection.HTTP_OK) {
System.out.println("------>responsecode" + 200 + "---------->" + musicname + "歌曲下载请求成功");
InputStream inputStream = httpURLConnection.getInputStream();
byte[] blist = new byte[1024];
File file = new File("C:\\Users\\23524\\Desktop\\music\\" + musicname + ".mp3");
FileOutputStream outputstream = new FileOutputStream(file);
int len = 0;
int progress = 0;
while ((len = inputStream.read(blist)) != -1) {
outputstream.write(blist, 0, len);
progress++;
float result = 0;
if ((progress % 512) == 0) {
System.out.println(progress / 512 * 0.5 + "MB");
}
}
float size = file.length() * 1.0f / 1024 / 1024;
System.out.println(musicname + "下载完毕,文件大小为" + (size - size % 0.01f) + "MB");
outputstream.flush();
outputstream.close();
inputStream.close();
} else {
System.out.println(httpURLConnection.getResponseCode() + "------->连接失败");
}
} catch (IOException ioe) {
ioe.printStackTrace();
}
}
public static void main(String[] args) throws UnsupportedEncodingException {
NetRequest_Music n = new NetRequest_Music();
// n.searchSongUrl("324243");
// n.downloadMusic("https://other-web-nf01-sycdn.kuwo.cn/d4f9fca65db317cf79b18366f6991699/62bf13e9/resource/n3/24/3/2525850365.mp3", "玫瑰花的葬礼");
// n.downloadMusic("https://other-web-rg01-sycdn.kuwo.cn/c6d9d08473569f74f20947f1f49164fd/62bf13ea/resource/n1/76/69/2539603307.mp3", "千古");
// n.getMusicList("徐良");
HashMap<String,String> listMap= n.getMusicList("许嵩");
Set<String> ks = listMap.keySet();
for (String k:ks
) {
NetRequest_Music.downloadMusic(n.searchSongUrl(k),listMap.get(k));
}
}
}代码如上,结果就是一条一条写爬取一首歌可以,但是一旦放到foreach中,就会
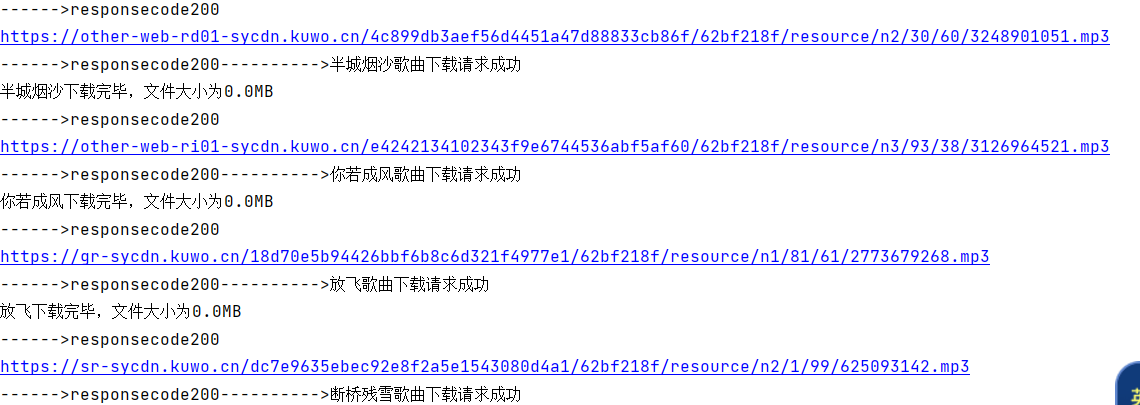
爬取一点,每个文件都只有两百多字节。求解答为什么。
打断点